To check if a set A is a superset of set B in Python, use the issuperset() method.
For instance:
A = {1, 2, 3, 4, 5, 6} B = {1, 2, 3} print(A.issuperset(B))
Result:
True
You can also use the built-in comparison operators to check for supersets in Python.
For instance, to check if set A is a superset of B, use the less than or equal >=
operator:
A = {1, 2, 3, 4, 5, 6} B = {1, 2, 3} print(A >= B)
Result:
True
A Word on Set Theory
Say you have two sets A and B.
- Set A is a superset of B if all the elements of set B are in set A.
- Set A set is always a superset of itself.
- Set A is a proper superset of B if set A is a superset of set B and the set A has elements B does not.
Here is a Venn diagram of set A being a (proper) superset of set B:
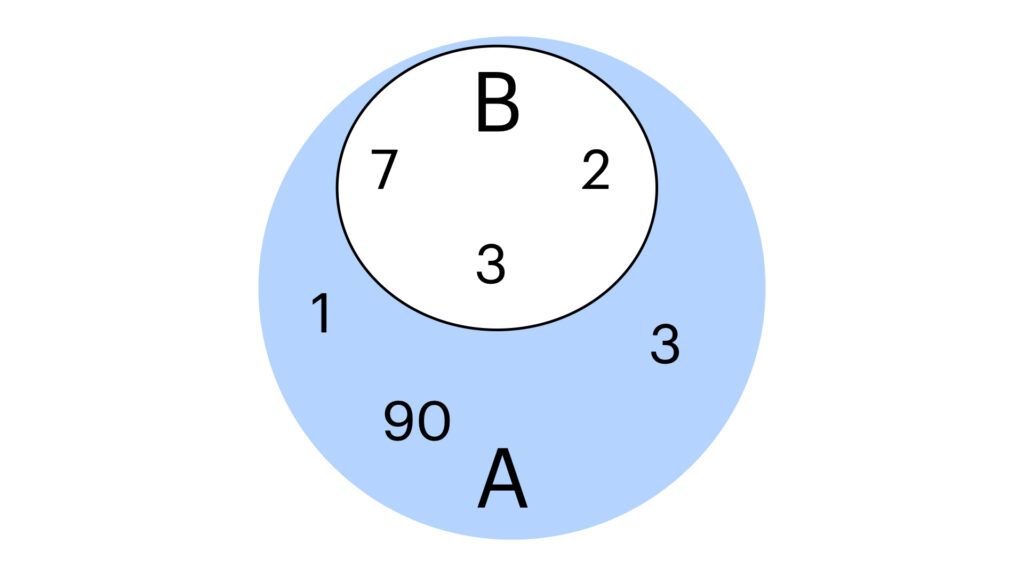
How to Check If a Set Is a Superset in Python
In Python, there is an issuperset()
method you can use to check if a set is a superset of another set.
Here is the syntax:
A.issuperset(B)
This returns True
if A
is a superset of B
. Otherwise, it returns False
.
Alternatively, you can also use comparison operators to do the same:
A >= B
For instance:
A = {1, 2, 3, 4, 5, 6} B = {1, 2, 3} print(A.issuperset(B)) # Or print(A >= B)
Result:
True True
A Set Is a Superset of Itself
A set is always a superset of itself.
You can use the issuperset()
method to verify this:
A = {1, 2, 3} print(A.issuperset(A)) # Or print(A >= A)
Output:
True True
How to Check If a Set Is a Proper Superset in Python
Set A is a proper superset of set B if all the elements of set B are in set A and set A has element(s) that set B does not have.
In Python, there is no dedicated method to check if a set is a proper subset of another set.
To check if a set is a proper superset of another set, use the less-than comparison operator:
A = {1, 2, 3, 4, 5, 6} B = {1, 2, 3} # Proper superset print(A > B)
Output:
True
Conclusion
Today you learned how to use issuperset()
method in Python. You also learned some basic set theory.
- Set A is a superset of set B if set A has all the elements of set B.
- Set A is a proper superset of B if all elements of set B are in set A but set A has at least one element that set B does not.
- A set is always a superset of itself.
In Python, you can check if set A is a superset of set B by:
- A.issuperset(B)
- A
>
= B
To check if a set A is a proper superset of set B:
- A > B
Thanks for reading. I hope you find it useful.
Happy coding!