To calculate the area of a circle in Python, here’s the code:
from math import pi def area(r): return pi * r ** 2
You can call this function by giving it the radius of the circle, for example:
print(area(10))
Output:
314.159....
How to Calculate the Area of a Circle in Maths
To calculate the area of the circle given the radius of the circle, use the following equation.
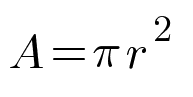
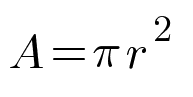
Sometimes you also have to calculate the area given the diameter or the circumference of the circle.
Without deriving these simple equations, here are all three formulas to calculate the area given:
- Radius
- Diameter
- Circumference
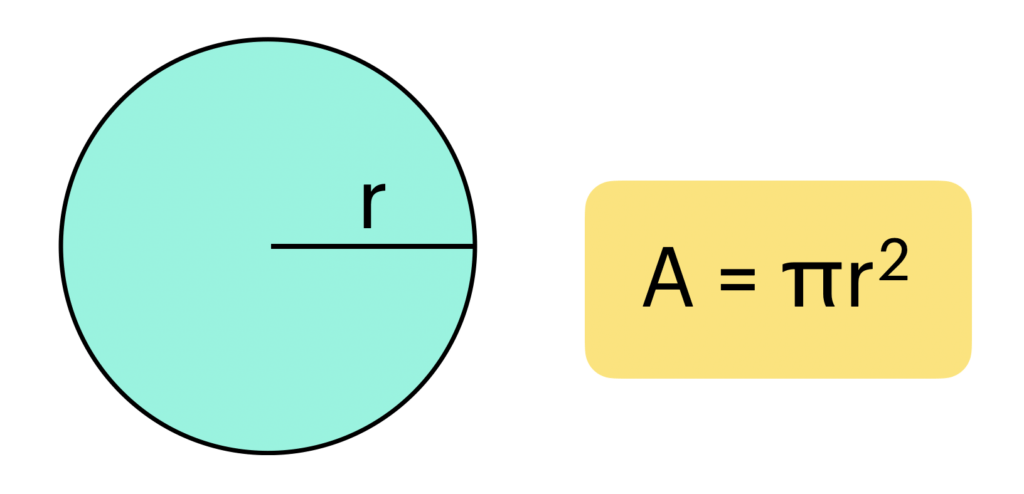
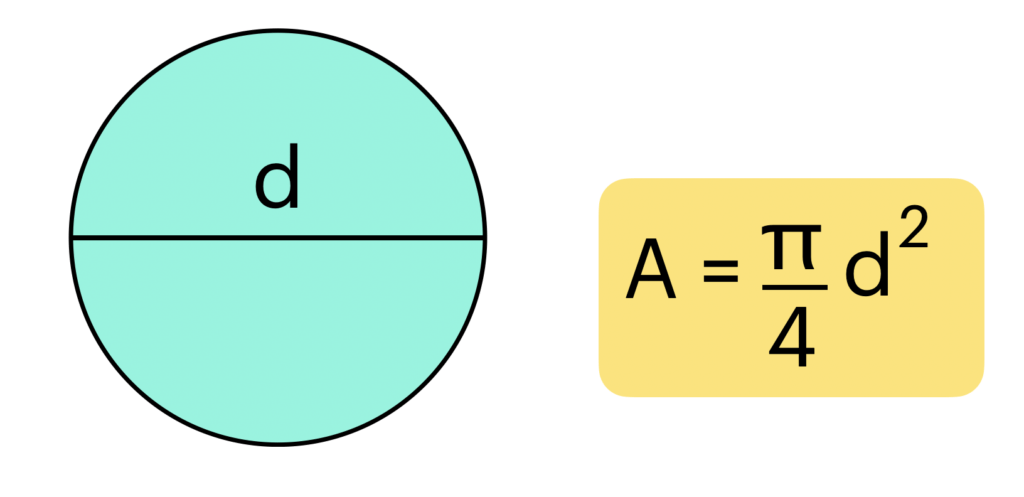
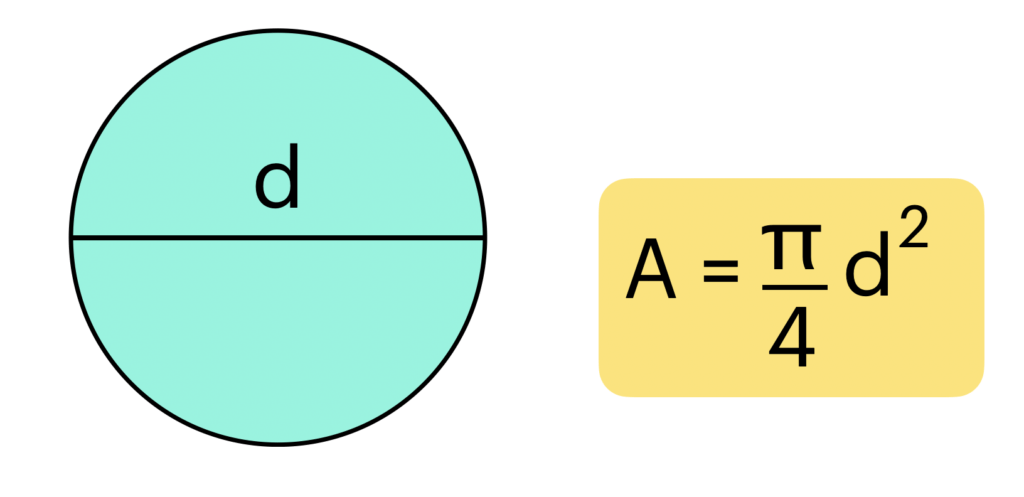
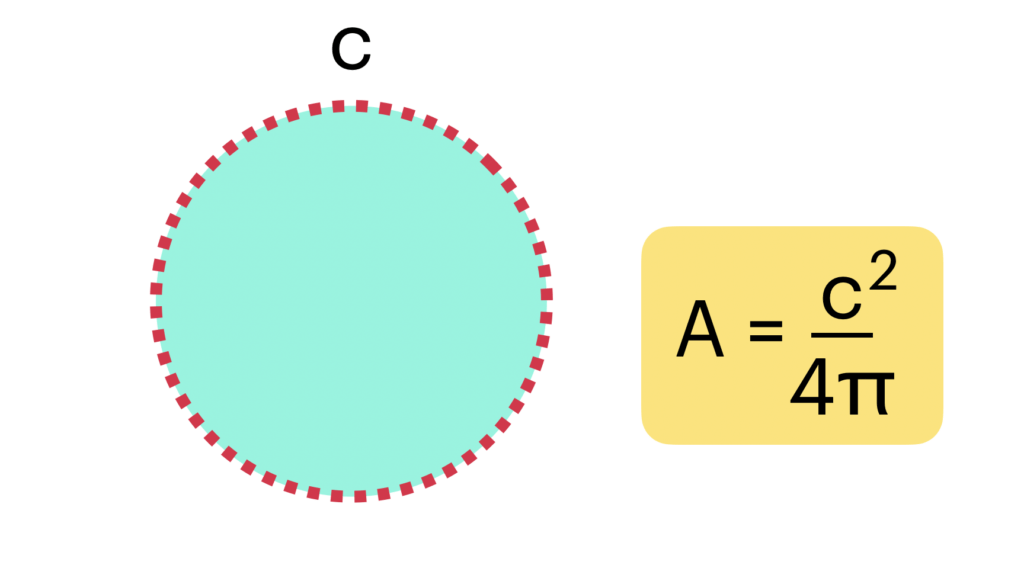
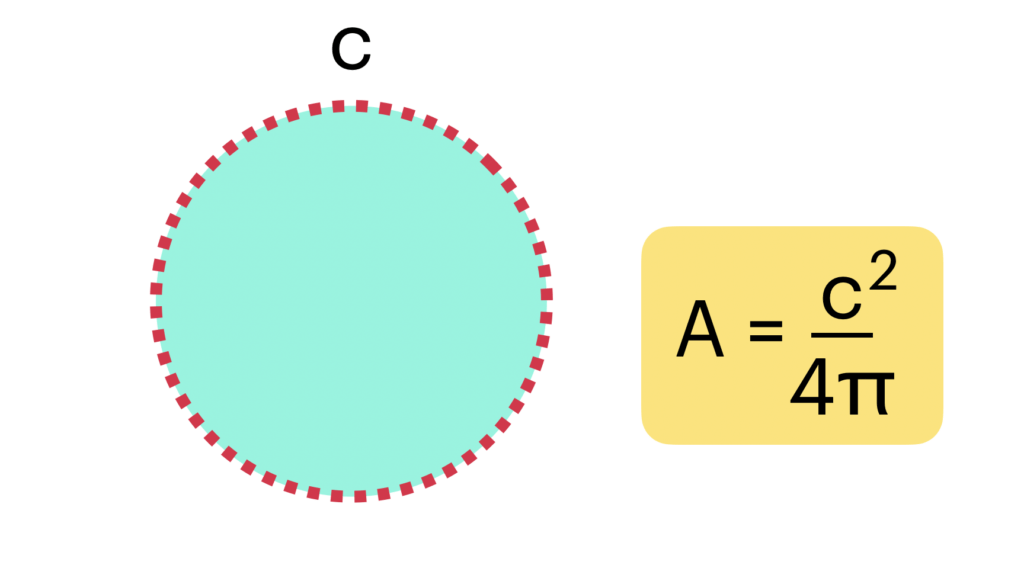
When you implement a Python program do the job using one of these formulas depending on what you want to accomplish with the program.
Program 1: Circle Area Given Radius
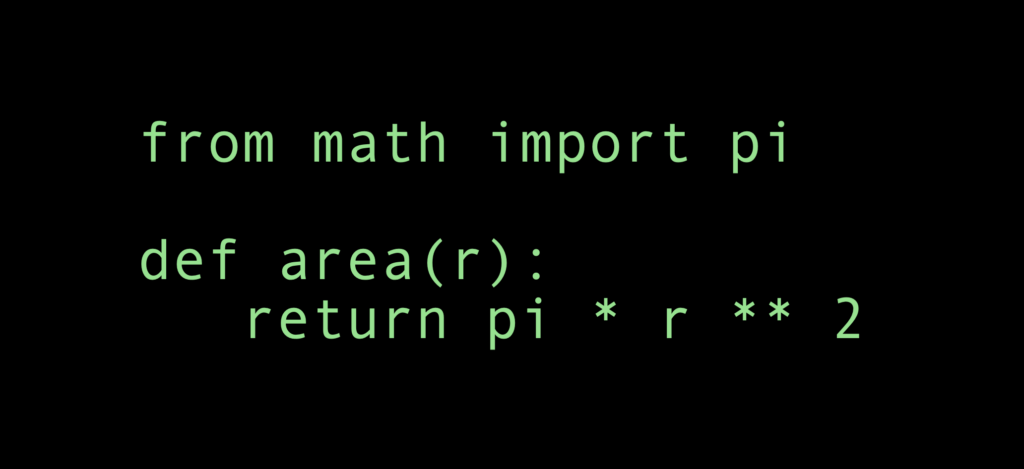
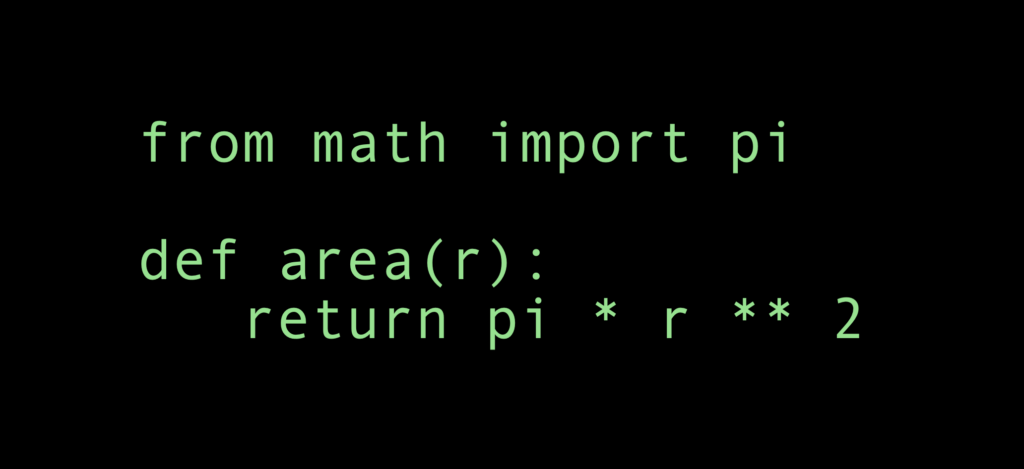
The area of a circle given the radius follows this formula:
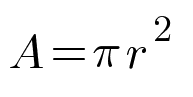
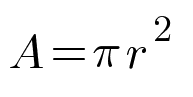
To convert this formula to a Python program, you need to:
- Create a function.
- Perform the calculation given the above formula.
- Return the result.
Here is the implementation for calculating the area of a circle given the circumference:
from math import pi def area(r): return pi * r ** 2
Program 2: Circle Area Given Diameter
The area of a circle given the diameter follows this formula:
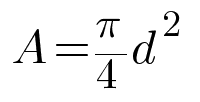
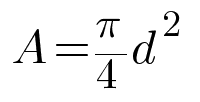
To convert this formula to a Python program, you need to:
- Create a function.
- Perform the calculation given the above formula.
- Return the result.
Here is the implementation for calculating the area of a circle given the circumference:
from math import pi def area(d): return (pi / 4) * d ** 2
Program 3: Circle Area Given Circumference
The area of a circle given the circumference follows this formula:
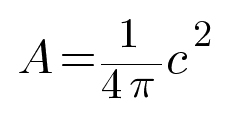
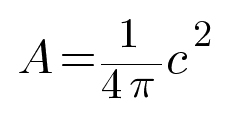
To convert this formula to a Python program, you need to:
- Create a function.
- Perform the calculation given the above formula.
- Return the result.
Here is the implementation for calculating the area of a circle given the circumference:
from math import pi def area(c): return (c ** 2) / (4 * pi)