Python zfill() method stretches a string by adding zeros at the beginning.
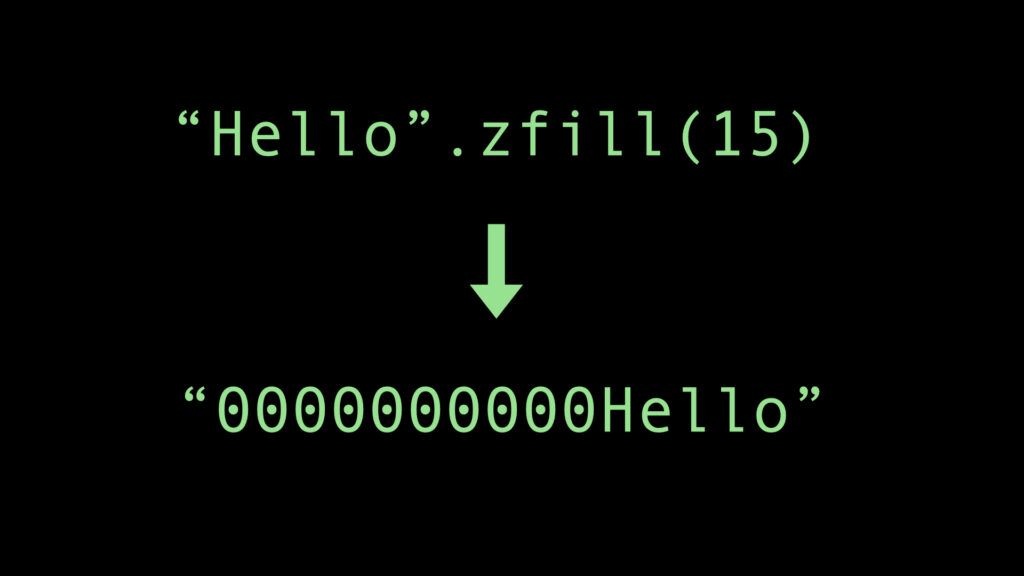
For instance, let’s add zeros to a string with zfill() until its length is 15:
sentence = "Hello world" print(sentence.zfill(15))
Output:
0000Hello world
This string is now 15
in length due to the zero-filling.
If the length of the string is greater than what is passed into the zfill()
, the method does nothing.
For example:
sentence = "Hello world" print(sentence.zfill(5))
Output:
Hello world
How Does It Work
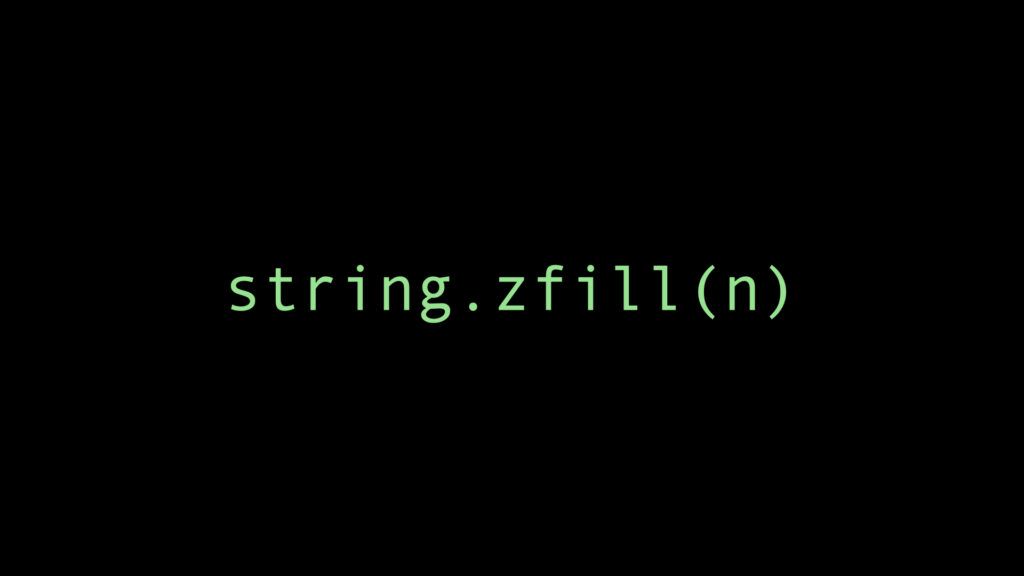
Syntax of the zfill
method:
string.zfill(n)
This method does not add n
zeros to the beginning. Instead, it makes the string n
in length by adding some number of zeros to it.
Also, the zfill()
method does not modify the original string. Instead, it returns a new string with zeros filled at the beginning of it.
Python zfill() Strings with a Leading +/- Sign
If a string starts with the prefix of + or -, the zfill()
method adds the zeros after the first occurrence of the prefix.
For example:
num_str1 = "-50" num_str2 = "+100" print(num_str1.zfill(10)) print(num_str2.zfill(10))
Output:
-000000050 +000000100
Or as another example:
word1 = "-test" word2 = "+hello" print(word1.zfill(10)) print(word2.zfill(10))
Output:
-00000test +0000hello
Python zfill() Strings with Multiple Leading +/- Signs
If there is more than one leading +
or -
sign in the string, the zeros are filled in after the first occurrence.
For example:
num_str1 = "--50" num_str2 = "++100" print(num_str1.zfill(10)) print(num_str2.zfill(10))
Output:
-000000-50 +00000+100
Python Zfill Right Side of a String
By default, the zfill()
method pads zeros at the beginning of the string (left side).
To zfill the string from the right in Python, you need to do a little trick:
Invert the string twice. Once before using the zfill()
and once after.
For example, let’s zfill a string from the right:
word = "Hello" print(word[::-1].zfill(10)[::-1])
Output:
Hello00000
Python zfill() Method Alternatives
Here are two alternatives to using zfill()
:
- The built-in
format()
method - string.rjust() method
1. Format() Method
The format() method can be used to embed variables into a string.
This means it can be used like the zfill()
method.
For example:
sentence = "Hello wolrd" print("{:0>15}".format(sentence)) print("{:0<15}".format(sentence))
Output:
0000Hello world
2. String.rjust() Method
String.rjust()
is a built-in string method. It stands for right-justified string. In other words, it adds a specific value to the left of the string until a specific string width is reached.
This method can be used to do the same as the zfill()
method.
For example:
sentence = "Hello world" print(sentence.rjust(15, '0'))
Output:
0000Hello world
Conclusion
Python’s built-in zfill() method pads a string by adding a number of zeros at the beginning of the string. It adds zeros until the desired string width is reached.
Thanks for reading. I hope you find it useful. Happy coding!